- Interview Preparation
- C# Interview Questions
- MVC Interview Questions
- Learning
- Learn C#
- Tools
- JSON Formatter & Minifier
- XML Formatter & Minifier
- CSS Formatter & Minifier
- SQL Formatter & Minifier
- Online Editors
- C# Editor
- HTML Editor
Top C# Interview Questions and Answers for 2025
Introduction
C# is one of the most popular programming language and was developed by Microsoft in 2000. It is simple yet effective and has an object-oriented programming concept that can be used for creating different types of applications. With the help of C# we can develop websites, web applications, mobile applications, cross platform web applications and many more.
In this article, we will provide 50+ C# Interview Questions and Answers which are suitable for freshers, intermediate and experienced professionals. These C# programming interview questions are written under the guidance of the masters of C# and by getting ideas through the experience of employees recent C# coding interviews.
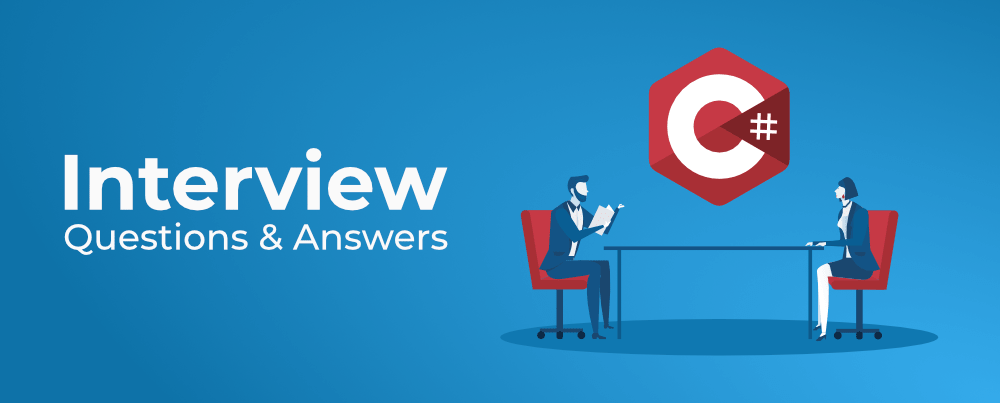
C# Interview Questions & Answers
1. What is C#?
C# is a powerful, versatile programming language developed by Microsoft. It's specifically designed for building a wide array of applications, ranging from desktop software to web applications and even mobile apps.
C# is known for its simplicity, robustness, and scalability, C# offers modern language features like object-oriented programming, strong typing, and automatic memory management through the Common Language Runtime (CLR). Its integration with the .NET framework provides access to a vast ecosystem of libraries and tools, making development efficient and productive.
Key features of C# include:
- Object-oriented: C# supports object-oriented programming concepts such as encapsulation, inheritance, and polymorphism.
- Type-safe: C# is a statically-typed language, which means that type checking is performed at compile-time, helping to catch errors early in the development process.
- Managed code: C# code is compiled into an intermediate language (IL) which is then executed by the Common Language Runtime (CLR). This provides benefits such as automatic memory management (garbage collection), exception handling, and security features.
- Modern language features: C# continues to evolve with new language features and enhancements, making it more expressive and efficient. Features like async/await, LINQ (Language Integrated Query), and pattern matching are some examples.
- Cross-platform development: With the introduction of .NET Core and later .NET 5 and .NET 6, C# has become increasingly cross-platform, allowing developers to build applications that run on Windows, Linux, and macOS.
- Rich ecosystem: C# benefits from a rich ecosystem of libraries, frameworks, and tools, including Visual Studio, Visual Studio Code, and various third-party libraries and frameworks for different types of applications.
Overall, C# stands as a cornerstone in modern software development, empowering developers to create high-quality, performant solutions across diverse platforms."
2. What is an object?
In C#, an object is an instance of a class. an object is possessing its own state (data) and behavior (methods). When you define a class in C#, you're essentially creating a blueprint for objects of that class type. The "New" keyword can be used to construct an object.
3. Define constructors with their types?
A constructor is a special method in a class that gets called when an instance of the class is created. It is used to initialize the object's state. In C#, constructors have the same name as the class and can be parameterized or parameterless.
4. What is boxing and unboxing in C#?
Boxing is the process of converting a value type (e.g., int, float) to a reference type (e.g., object) so that it can be stored on the heap.
Unboxing is the process of converting a reference type (e.g., object) back to a value type. It involves extracting the value from the boxed object.
5. What is Managed or Unmanaged Code?
Managed code in C# runs within a managed environment like the Common Language Runtime (CLR), providing automatic memory management and security features. Unmanaged code, however, operates outside of such environments, directly interacting with system resources and requiring manual memory management.
6. What is the difference between a struct and a class in C#?
Class and struct are both user-defined data types but have some major differences: Struct The struct is a value type in C# and inherits from System.Value Type. Struct is usually used for smaller amounts of data. Struct can’t be inherited from other types. A structure can't be abstract. No need to create an object with a new keyword. Do not have permission to create any default constructor. Class The class is a reference type in C#, and it inherits from the System.Object Type. Classes are usually used for large amounts of data. Classes can be inherited from other classes. A class can be an abstract type. We can create a default constructor.
7. What is the difference between a value type and a reference type in C#?
A value type stores its data directly, while a reference type stores a reference (memory address) to the location where its data is stored. Value types are typically primitive types like int, float, bool, etc., while reference types are usually classes, interfaces, delegates, or arrays. Value types are stored on the stack, while reference types are stored on the heap.
8. What is the purpose of the using statement in C#?
The 'using' statement is used to ensure that the resources like file streams, database connections, etc., are properly disposed of when they are no longer needed. It automatically calls the Dispose method of IDisposable interface when the block of code is exited.
9. What is the difference between ref and out parameters in C#?
The 'ref' keyword is used to pass arguments to a method by reference, meaning any changes to the parameter inside the method will reflect in the calling code.
The 'out' keyword is similar to 'ref', but it is used when a method returns multiple values. It indicates that the parameter is being used to return a value from the method.
10. What is an enum in C#?
In C#, an enum (enumeration) is a value type that allows you to define a set of named constants. It provides a way to associate meaningful names with a set of related integral values, making the code more readable and maintainable. Enums are often used to represent a fixed set of possible values for a variable or parameter.
Here's an example of defining and using an enum in C#:
// Define an enum named "DaysOfWeek" with Monday as 1, Tuesday as 2, and so on.
public enum DaysOfWeek
{
Monday = 1,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday
}
class Program
{
static void Main(string[] args)
{
// Assigning enum values to variables
DaysOfWeek today = DaysOfWeek.Tuesday;
Console.WriteLine("Today is: " + today); // Output: Today is: Tuesday
// Enum iteration
foreach (DaysOfWeek day in Enum.GetValues(typeof(DaysOfWeek)))
{
Console.WriteLine(day);
}
}
}
11. What are the different type of loops in C#?
In C#, there are several types of loops that allow us to execute a block of code repeatedly until a certain condition is met. The main types of loops are:
for loop: The 'for' loop is used to iterate over a range of values or elements in a collection. It consists of an initialization statement, a condition, and an iteration statement. The loop continues executing as long as the condition evaluates to true.
while loop: The 'while' loop is used to execute a block of code repeatedly as long as a specified condition is true. It evaluates the condition before executing the loop body, so it's possible that the loop body may never execute if the condition is initially false.
do-while loop: Similar to the 'while' loop, the 'do-while' loop also executes a block of code repeatedly based on a condition. However, it evaluates the condition after executing the loop body, ensuring that the loop body is executed at least once before checking the condition.
foreach loop: The 'foreach' loop is used to iterate over elements in a collection, such as arrays, lists, or other enumerable types. It simplifies the process of iterating through each element of a collection by automatically handling the iteration logic.
12. What is the difference between “continue” and “break” statements in C#?
'continue' is used to skip the rest of the loop's body for the current iteration and proceed to the next iteration. It allows the loop to continue with the next iteration, ignoring the remaining code in the loop's body for the current iteration
'break'is used to terminate the loop prematurely and exit its execution. It immediately exits the loop, bypassing any remaining iterations, and continues with the code outside the loop.
Example using 'break':
using System;
class Program
{
static void Main(string[] args)
{
// Break out of the loop when 'i' reaches 5
for (int i = 1; i <= 10; i++)
{
if (i == 5)
{
Console.WriteLine("Breaking out of the loop at i = 5");
break;
}
Console.WriteLine("Current value of i: " + i);
}
}
}
Output:
Current value of i: 1
Current value of i: 2
Current value of i: 3
Current value of i: 4
Breaking out of the loop at i = 5
Example using 'continue':
using System;
class Program
{
static void Main(string[] args)
{
// Skip printing even numbers using 'continue'
for (int i = 1; i <= 10; i++)
{
if (i % 2 == 0)
{
// Skip printing even numbers
continue;
}
Console.WriteLine("Current value of i: " + i);
}
}
}
Output:
Current value of i: 1
Current value of i: 3
Current value of i: 5
Current value of i: 7
Current value of i: 9
13. What is the difference between constant and readonly in C#?
In C#, constant and readonly are both used to declare variables whose values cannot be changed after initialization, but they have some key differences.
constant | readonly |
'const' keyword is use to declare the constant value | 'readonly' keyword is use to declare the readonly value |
'const' is a compile-time constant and must be initialized with a value at the time of declaration | 'readonly' allows a value to be assigned either at the time of declaration or within the constructor of the containing class |
its value cannot be changed throughout the program | its value can be changed only within the constructor |
'const' variables are implicitly static | 'readonly' variables are not. |
14. What is the async and await keywords used for in C#?
'async' is used to define a method that can perform asynchronous operations. 'await' is used inside an 'async' method to indicate that the method should asynchronously wait for the completion of the awaited task before proceeding.
15. What are the access modifiers available in C# and what do they mean?
Access modifiers control the accessibility of classes, methods, properties, and other members in C#. There are five access modifiers:
- public: Accessible from any other code.
- private: Accessible only within the containing class.
- protected: Accessible within the containing class and derived classes.
- internal: Accessible within the same assembly.
- protected internal: Accessible within the same assembly or from derived classes in other assemblies.
16. What is the difference between value types and reference types in C#?
Value types: directly contain their data and are stored in the stack memory. Examples include int, float, enum, struct, etc. Reference types: store a reference to the actual data in memory and are stored on the heap. Examples include class, interface, delegate, string, etc.
17. Explain the difference between String and StringBuilder in C#?
'String' is immutable, meaning its value cannot be changed after it's created. Each manipulation creates a new string object. 'StringBuilder' is mutable and is designed for scenarios where strings are frequently manipulated. It provides methods to modify its contents without creating new objects, thus improving performance.
18. What are the Arrays in C#?
Arrays in C# are data structures that allow you to store multiple elements of the same type. Arrays can be a fixed or dynamic in size. Arrays used index to retrive the data and it always starts with 0.
Fixed size arrays can store a predefined number of items and determined at the time of declaration, and elements can be accessed using an index. You cannot the change the fixed size to dynamic array.
Basic example of fixed size array:
int[] numbers = new int[5];
In above example, we have declared 'numbers' array with fixed size of 5 elements.
Dynamic arrays can store any number of items and it does not have any predefined size. It dynamically increased the array size as you add the item. You can declare an array of fixed length or dynamic. You can even change a dynamic array to static after it is defined.
19. What is a Jagged Array in C#?
A jagged array is an array whose elements can be arrays. It allow each row (or element) to have a different length or size, providing more flexibility in data storage. A jagged array also known as array of arrays.
Here's an example of a jagged array:
int[][] jaggedArray = new int[3][];
// Assigning arrays of different lengths to each element
jaggedArray[0] = new int[] { 1, 2, 3 }; // Array with 3 elements
jaggedArray[1] = new int[] { 4, 5 }; // Array with 2 elements
jaggedArray[2] = new int[] { 6, 7, 8, 9 }; // Array with 4 elements
In this example, jaggedArray is a jagged array with 3 elements, where each element is an integer array of varying lengths. The lengths of the arrays assigned to each element can differ, giving the jagged array its flexibility.
20. What are the differences between ArrayList and List in C#?
Both ArrayList and List
ArrayList | List |
ArrayList is a non-generic collection class | List |
ArrayList can hold elements of any data type | List |
ArrayList resizes dynamically when elements are added or removed, which can impact performance due to boxing and unboxing operations | List |
ArrayList is a legacy collection class that lacks type safety and is less efficient | List |
21. What is the difference between const and readonly in C#?
'const' is used to declare constants, which are values that cannot be changed after initialization. They are implicitly static and must be initialized at the time of declaration.
'readonly' is used to declare fields that can only be assigned a value once, either at the time of declaration or in a constructor. They are not constants but rather fields whose values cannot be changed after initialization.
22. What is the purpose of the yield keyword in C#?
The 'yield' keyword is used in iterator methods to provide a simple way to implement custom iterators. It enables deferred execution of a sequence of values, allowing the caller to consume the elements one at a time as needed. Here's an example:
public IEnumerable GenerateSequence()
{
for (int i = 0; i < 10; i++)
{
yield return i;
}
}
23. What’s the difference between the System.Array.CopyTo() and System.Array.Clone()?
The difference between System.Array.CopyTo() and System.Array.Clone() lies in their functionalities and how they handle array copying in C#:
System.Array.CopyTo():
CopyTo() is a method provided by the System.Array class.
It allows you to copy the elements of one array to another existing array.
The target array must already exist and have sufficient space to accommodate the copied elements. If the target array is not large enough, an exception will be thrown.
The elements are copied to the target array starting at the specified index.
It performs a shallow copy, meaning that if the elements of the source array are reference types, only the references to the objects are copied, not the objects themselves.
It does not create a new array instance but instead modifies an existing array.
System.Array.Clone():
Clone() is a method provided by the System.Array class.
It creates a shallow copy of the array, including the same number of elements and the same values.
The copied array is a separate instance from the original array.
It does not require a target array to be specified, as it creates a new array instance.
Similar to CopyTo(), it performs a shallow copy, meaning that if the elements of the source array are reference types, only the references to the objects are copied, not the objects themselves.
It returns an object of type System.Object, so you need to cast it back to the appropriate array type.
24. What are the different ways to handle errors in C#?
Error handling in C# can be done using:
Try-catch blocks to catch and handle exceptions. Throw statements to manually throw exceptions. Finally blocks to execute cleanup code regardless of whether an exception occurs. Exception filters to catch specific exceptions based on conditions. Global exception handling using AppDomain or application-level event handlers.
25. List down the most commonly used types of exceptions in C#
In C#, several commonly used types of exceptions are:
- System.NullReferenceException: Occurs when you try to access a member on a null object reference.
- System.ArgumentNullException: Thrown when a null argument is passed to a method that doesn't accept it.
- System.ArgumentException: Raised when one or more arguments passed to a method are invalid.
- System.InvalidOperationException: Indicates that a method call is not valid for the object's current state.
- System.IO.IOException: Thrown for I/O-related issues, such as file not found or access denied.
- System.FormatException: Occurs when a format of an argument is not compatible with the expected format.
- System.ArithmeticException: Represents errors in arithmetic operations, such as division by zero.
- System.NotSupportedException: Indicates that a method or operation is not supported.
- System.OutOfMemoryException: Thrown when there's not enough memory to continue the execution.
- System.IndexOutOfRangeException/System.ArgumentOutOfRangeException: Indicates that an index or argument is outside the range of valid values.
These are just a few examples; there are many more specific exception types available in the .NET Framework for handling various error conditions.
26. Can Multiple Catch Blocks be executed in C#?
No, in C#, only one catch block is executed for any given exception. When an exception is thrown, the runtime searches for the first catch block that matches the type of the thrown exception or one of its base types. Once a matching catch block is found, its associated code is executed, and subsequent catch blocks are skipped. This ensures that only one catch block handles the exception, promoting clarity and predictability in exception handling.
27. What are Custom Exceptions?
Custom exceptions in C# allow developers to create their own exception types to represent specific error conditions or situations within their applications. By deriving custom exception classes from the base Exception class or one of its derived classes, developers can provide more meaningful error messages and handle exceptional situations more effectively.
28. What is the purpose of the lock keyword in C#?
The lock keyword in C# serves the purpose of synchronizing access to a shared resource or critical section of code in a multi-threaded environment. Its primary function is to prevent multiple threads from concurrently executing a block of code that modifies shared data, thereby avoiding race conditions and ensuring thread safety.
When you use the lock keyword, you specify an object as a synchronization token. This object is used to establish a mutual exclusion lock. Only one thread can obtain the lock for that object at a time. Other threads that try to acquire the lock will be blocked until the lock is released by the thread that acquired it.
Here's a basic example of how lock is used:
class Example
{
private readonly object _lock = new object();
private int _sharedData = 0;
public void ModifySharedData()
{
lock (_lock)
{
// Critical section: Access shared data
_sharedData++;
}
}
}
In this example, the lock statement ensures that only one thread can execute the critical section (incrementing _sharedData) at a time. This prevents potential issues such as data corruption or inconsistent state due to concurrent access by multiple threads.
29. What are nullable types in C#?
Nullable types in C# allow variables to have a "null" stored in addition to their normal range of values. They are represented by adding a "?" after the type declaration. For example, int? denotes a nullable integer. Nullable types are particularly useful when dealing with database values, where a field might be empty.
Basic example of nullable types:
int? nullableInt = null;
double? nullableDouble = 3.14;
bool? nullableBool = true;
30. What is the difference between == and Equals() method in C#?
==is used to compare the value of two operands. For value types, it compares the actual values, while for reference types, it compares the references. Equals() is a method inherited from the Object class and is used to compare the contents of two objects. It can be overridden in derived classes to provide custom comparison logic.
31. What is a lambda expression in C#?
A lambda expression in C# allows you to define anonymous functions. It allows you to create functions inline without explicitly declaring a separate method. Lambda expressions are commonly used in LINQ queries, event handling, and functional programming scenarios to write more expressive and readable code.
Here's a simple example of a lambda expression:
Func add = (a, b) => a + b;
In this example, (a, b) => a + b is a lambda expression that represents an anonymous function taking two integers a and b as parameters and returning their sum. The Func
32. What is the difference between IEnumerable and IQueryable in C#?
'IEnumerable' is used to represent a collection of objects that can be enumerated. It is suitable for in-memory collections and works with LINQ to Objects. 'IQueryable' is used to represent a query that can be executed against a specific data source, such as a database. It works with LINQ to SQL, LINQ to Entities, etc., allowing the query to be translated into SQL or other query languages for execution on the data source.
33. What is serialization in c#?
Serialization in C# refers to the process of converting an object into a format that can be easily stored, transmitted, or reconstructed at a later time. It involves converting the state of an object into a stream of bytes. The reverse process is called deserialization, reconstructing an object from its serialized form.
Serialization is commonly used in various scenarios, such as:
- Persisting object state to a file or database for later retrieval.
- Sending objects over a network in distributed systems or web services.
- Sharing data between different applications or platforms.
- Caching objects to improve application performance.
C# offers various serialization techniques:
- XML Serialization: This method serializes objects into XML format, making it readable and human-editable. It's commonly used for configuration files, interoperability, and data exchange between different platforms.
- Binary Serialization: Binary serialization converts objects into binary format, which is more compact and efficient than XML. It's faster and suitable for scenarios where performance is critical, such as saving objects to disk or transmitting data over a network.
- JSON Serialization: JSON (JavaScript Object Notation) serialization is used to convert objects into JSON format, which is lightweight and widely supported in web development. It's commonly used in web APIs and AJAX requests.
34. What is method overloading and method overriding in C#?
Method overloading is a feature that allows a class to have multiple methods with the same name but different parameters. The compiler determines which method to call based on the number and types of arguments passed.
Method overriding is a feature that allows a derived class to provide a specific implementation of a method that is already defined in its base class. It enables polymorphism and dynamic method invocation
35. What are the Different Ways a Method can be Overloaded?
In C#, a method can be overloaded in several ways to provide multiple implementations with different parameter lists. Here are the different ways a method can be overloaded:
Different Number of Parameters:
You can define multiple methods with the same name but different numbers of parameters. For example:
void Method(int a);
void Method(int a, int b);
void Method(int a, int b, int c);
Different Data Types of Parameters:
Overloaded methods can have the same number of parameters, but of different data types. For example:
void Method(int a);
void Method(double a);
Different Order of Parameters:
The order of parameters can also be different between overloaded methods. For example:
void Method(int a, double b);
void Method(double b, int a);
Optional Parameters:
You can define methods with optional parameters. In this case, the method can be called with different numbers of arguments. For example:
void Method(int a, int b = 0);
void Method(int a, int b, int c = 0);
Different Return Types:
Overloaded methods can have the same parameter list but different return types. However, the return type alone is not sufficient to overload a method. You must also have different parameter types or a different number of parameters. For example:
int Method(int a);
double Method(int a);
36. What is an Object Pooling?
Object Pooling in .NET allows objects to be kept in the memory pool so they can be reused without recreating them. The primary purpose of Object Pooling is to minimize the overhead associated with object creation and destruction, particularly in scenarios where creating objects is resource-intensive or time-consuming.
Object Pooling is commonly used in scenarios such as database connections, network connections, threads, and other resources that are expensive to create. By reusing objects from the pool, the application can improve performance, reduce memory fragmentation, and minimize the impact of garbage collection.
Object Pooling typically involves the following steps:
- Initialization: Create a pool of objects and initialize them to a valid state.
- Acquisition: When an object is needed, acquire it from the pool. If no objects are available, a new one may be created if allowed.
- Usage: Use the acquired object for the desired task.
- Release: Once the object is no longer needed, release it back to the pool for reuse.
37. What is the difference between Task and Thread in C#?
In C#, both Task and Thread are mechanisms for executing code asynchronously, but they have significant differences in terms of abstraction, manageability, and overhead:
Task | Thread |
Higher-level abstraction | Lower-level abstraction |
Managed by .NET ThreadPool | Managed by operating system kernel |
Scheduled asynchronously | Synchronous execution |
More lightweight | More heavyweight |
Supports cancellation, continuations, etc. | Limited features and control |
Built-in support for exception handling | Error handling can be challenging |
Used for asynchronous operations | Used for low-level CPU-bound operations |
38. What are Generics in C#?
Generics in C# provide a way to create classes, structures, methods, and interfaces that operate with unspecified data types while still maintaining type safety at compile time. They allow you to define reusable, type-parameterized components that can work with any data type, thus enhancing code flexibility and reusability.
The key benefits of generics include:
- Type Safety: Generics enable type-safe operations by allowing you to specify the data type(s) a generic type or method will operate on. This ensures that type mismatches are caught at compile time rather than runtime, reducing the likelihood of errors.
- Code Reusability: With generics, you can write code that works with any data type, eliminating the need to duplicate logic for each specific data type. This promotes code reusability and minimizes redundancy in your codebase.
- Performance: Generics can improve performance by avoiding boxing and unboxing operations, which occur when converting value types to reference types. By using generics, you can work directly with value types, leading to more efficient code execution.
- Maintainability: Generic code tends to be more maintainable and easier to understand because it promotes a higher level of abstraction. By creating components that operate independently of specific data types, you can build more modular and flexible applications.
using System;
using System.Collections.Generic;
public class GenericStack
{
private List items = new List();
public void Push(T item)
{
items.Add(item);
}
public T Pop()
{
if (items.Count == 0)
throw new InvalidOperationException("Stack is empty");
T item = items[items.Count - 1];
items.RemoveAt(items.Count - 1);
return item;
}
}
class Program
{
static void Main(string[] args)
{
// Create a stack of integers
GenericStack intStack = new GenericStack();
intStack.Push(1);
intStack.Push(2);
intStack.Push(3);
Console.WriteLine("Popping integers from the stack:");
Console.WriteLine(intStack.Pop()); // Output: 3
Console.WriteLine(intStack.Pop()); // Output: 2
Console.WriteLine(intStack.Pop()); // Output: 1
// Create a stack of strings
GenericStack stringStack = new GenericStack();
stringStack.Push("Hello");
stringStack.Push("World");
Console.WriteLine("Popping strings from the stack:");
Console.WriteLine(stringStack.Pop()); // Output: World
Console.WriteLine(stringStack.Pop()); // Output: Hello
}
}
In this example, the GenericStack
using System;
// Custom exception class for representing invalid input errors
public class InvalidInputException : Exception
{
public InvalidInputException() { }
public InvalidInputException(string message) : base(message) { }
public InvalidInputException(string message, Exception innerException) : base(message, innerException) { }
public InvalidInputException(string message, string additionalInfo) : base(message)
{
AdditionalInfo = additionalInfo;
}
public string AdditionalInfo { get; }
}
public class Calculator
{
public int Divide(int dividend, int divisor)
{
if (divisor == 0)
{
throw new InvalidInputException("Cannot divide by zero", "Divisor: " + divisor);
}
return dividend / divisor;
}
}
class Program
{
static void Main(string[] args)
{
Calculator calculator = new Calculator();
try
{
int result = calculator.Divide(10, 0);
Console.WriteLine($"Result: {result}");
}
catch (InvalidInputException ex)
{
Console.WriteLine($"Invalid input error: {ex.Message}");
Console.WriteLine($"Additional Info: {ex.AdditionalInfo}");
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
In this example, the InvalidInputException class is a custom exception derived from Exception, designed to represent errors related to invalid input. The Calculator class throws this custom exception when attempting to divide by zero. The Main method demonstrates catching and handling the custom exception, including accessing additional information provided by the exception.
39. What is the purpose of the finally block in C# exception handling?
The finally block in C# exception handling serves the purpose of providing a section of code that is guaranteed to execute, regardless of whether an exception occurs or not. It ensures cleanup or resource release operations are performed, making it particularly useful for tasks such as closing files, releasing database connections, or disposing of objects.
using System;
using System.IO;
class Program
{
static void Main()
{
FileStream fileStream = null;
try
{
fileStream = File.Open("example.txt", FileMode.Open);
// Perform some operations with the file
// Simulate an exception
throw new IOException("An error occurred while processing the file");
}
catch (IOException ex)
{
Console.WriteLine("An IOException occurred: " + ex.Message);
}
finally
{
// Ensure that the file stream is closed, regardless of whether an exception occurred or not
fileStream?.Close();
}
}
}
In this example, the finally block ensures that the fileStream is closed, even if an IOException is thrown within the try block. This prevents resource leaks and ensures proper cleanup of the file stream, maintaining code integrity.
40. What is a Virtual Method in C#?
A virtual method in C# is a method that is declared in a base class and can be overridden (redefined) in derived classes. It enables polymorphism, allowing objects of different derived classes to provide their own implementation of the same method signature defined in the base class.
using System;
// Base class with a virtual method
public class Shape
{
public virtual void Draw()
{
Console.WriteLine("Drawing a shape");
}
}
// Derived class that overrides the virtual method
public class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a circle");
}
}
// Another derived class that overrides the virtual method
public class Rectangle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a rectangle");
}
}
class Program
{
static void Main(string[] args)
{
// Create objects of different derived classes
Shape shape1 = new Circle();
Shape shape2 = new Rectangle();
// Call the overridden method
shape1.Draw(); // Output: Drawing a circle
shape2.Draw(); // Output: Drawing a rectangle
}
}
In this example, the Shape class declares a virtual method Draw(). The Circle and Rectangle classes override this method with their own implementations. When calling the Draw() method on objects of type Circle and Rectangle, the appropriate overridden method is executed based on the actual type of the object, demonstrating polymorphic behavior.
41. What are sealed classes in C#?
The sealed class is used to prevent inheritance of a class or override of a method. When a class is marked as sealed, it cannot be used as a base class for other classes. When a method is marked as sealed, it cannot be overridden in derived classes.
42. What is meant by an Abstract Class?
An abstract class in C# is a class that cannot be instantiated directly and serves as a blueprint for other classes. It may contain abstract methods, which are declared without implementation and must be implemented by derived classes.
Abstract classes can also contain concrete methods, fields, properties, and events. They provide a way to define common behavior and structure for a group of related classes, while allowing specific implementations to be defined by subclasses.
Abstract classes are often used to define common interfaces or base functionality in inheritance hierarchies.
43. What is polymorphism in C#?
Polymorphism is a concept of object-oriented programming that refers to the ability of a single interface to be used to refer to multiple implementations of a particular behavior. In C#, polymorphism can be achieved through inheritance, interfaces, and virtual methods.
This concept enables code flexibility and reusability by allowing different classes to be treated uniformly based on their common base type.
There are two main types of polymorphism in C#: compile-time polymorphism and runtime polymorphism.
Compile-time polymorphism
Compile-time is achieved through method overloading and operator overloading, where multiple methods or operators can have the same name but different parameters or behaviors.
Here's an example
using System;
public class MathOperations
{
// Method overloading
public int Add(int a, int b)
{
return a + b;
}
public double Add(double a, double b)
{
return a + b;
}
// Operator overloading
public static MathOperations operator +(MathOperations op1, MathOperations op2)
{
MathOperations result = new MathOperations();
result.Value = op1.Value + op2.Value;
return result;
}
public int Value { get; set; }
}
class Program
{
static void Main(string[] args)
{
MathOperations mathObj = new MathOperations();
// Method overloading example
int sumIntegers = mathObj.Add(3, 5);
double sumDoubles = mathObj.Add(3.5, 2.7);
Console.WriteLine($"Sum of integers: {sumIntegers}"); // Output: Sum of integers: 8
Console.WriteLine($"Sum of doubles: {sumDoubles}"); // Output: Sum of doubles: 6.2
// Operator overloading example
MathOperations obj1 = new MathOperations { Value = 5 };
MathOperations obj2 = new MathOperations { Value = 3 };
MathOperations result = obj1 + obj2;
Console.WriteLine($"Sum of objects: {result.Value}"); // Output: Sum of objects: 8
}
}
Runtime polymorphism
Runtime polymorphism, on the other hand, is achieved through method overriding and interface implementation. In method overriding, a method in the derived class overrides a method in the base class with the same signature, allowing for different implementations of the same method.
44. What is inheritance in C#?
Inheritance is a mechanism in C# by which a new class can be derived from an existing class. The derived class inherits all the members (fields, properties, methods) of the base class. It promotes code reuse and supports the concept of polymorphism.
45. Is it possible for a private virtual method to be overridden?
No, it is not possible for a private virtual method to be overridden in C#. The 'private' access modifier restricts access to the method to the containing class only, making it inaccessible to derived classes. Therefore, even if the method is declared as 'virtual', which allows for overriding in derived classes, it cannot be overridden because it cannot be accessed outside the containing class.
46. What is the difference between Interface and Abstract Class in C#?
An abstract class can contain concrete methods along with abstract methods. It can also have fields and constructors. It cannot be instantiated directly and must be inherited. An interface can only contain method signatures, properties, events, and indexers. It cannot have any implementations. A class can implement multiple interfaces, but it can inherit only one abstract class.
47. What are partial classes in C#? Why do we need partial classes?
REVIEW A partial class is a feature in C# that allows a class's definition to be split into multiple files. Each part of the class is declared with the 'partial' keyword. This feature is commonly used in code generation scenarios and to organize large classes into manageable parts.
48. What are extension methods in C#?
Extension methods allow you to add new methods to existing types without modifying the original type or creating a new derived type. They are defined as static methods in a static class and are invoked as if they were instance methods of the extended type. Extension methods are commonly used to add functionality to .NET framework types or third-party libraries.
public static class StringExtensions
{
public static bool IsPalindrome(this string str)
{
// Implementation to check if the string is a palindrome
}
}
// Usage:
string text = "racecar";
bool isPalindrome = text.IsPalindrome();
49. What is a delegate in C#?
A delegate is a type that represents references to methods with a particular signature. It is similar to a function pointer in C++. Delegates are used to implement callback functions, event handling, and asynchronous programming in C#.
50. Explain the concept of garbage collection in C#
Garbage collection is a process in .NET where the runtime automatically manages memory by reclaiming objects that are no longer in use. It identifies and releases memory occupied by objects that are no longer reachable, allowing it to be reused by the application.
51. What is LINQ in C#?
LINQ is stands for Language Integrated Query. It enables you to querying data from different sources such as collections, arrays, databases, XML, and more using a uniform syntax, similar to SQL queries. LINQ provides powerful capabilities for filtering, sorting, grouping, and projecting data, making it easier and more efficient to work with data in C# applications.
52. What are lambda expressions in C#?
Lambda expressions are a concise way to represent anonymous methods in C#. They allow you to define a method without explicitly declaring a separate named method. Lambda expressions are often used in LINQ queries, event handling, and asynchronous programming.
53. What is the difference between FirstOrDefault() and First() methods in LINQ?
In LINQ, both FirstOrDefault() and First() methods are used to retrieve the first element from a sequence. However, there is a difference in behavior:
FirstOrDefault() | First() |
Returns the first element of the sequence or a default value if the sequence is empty. | Returns the first element of the sequence. |
Does not throw an exception if the sequence is empty. | Throws an exception (InvalidOperationException) if the sequence is empty. |
Useful when you expect the sequence may not contain any elements, and you want to handle the possibility of an empty sequence gracefully. | Suitable when you are confident that the sequence will always contain at least one element, and you want to retrieve it without checking the sequence's emptiness explicitly. |
In summary, FirstOrDefault() is more forgiving and returns a default value when the sequence is empty, while First() is more strict and throws an exception in such cases. Use FirstOrDefault() when dealing with the possibility of an empty sequence, and First() when you're certain that the sequence contains at least one element.
54. What is Reflection in C#?
Reflection is the ability of a program to examine its own structure, metadata, and behavior at runtime. It allows you to inspect and manipulate types, methods, properties, and other members dynamically.
55. What is an event in C#?
An event in C# is a mechanism that enables a class to notify other classes or objects when something of interest occurs. It is based on the publisher-subscriber design pattern. Events are declared using delegates and can be raised (or fired) by the publisher class.
56. Explain the concept of delegates multicast in C#
Delegates in C# support multicast invocation, which means that a single delegate instance can hold references to multiple methods and invoke them sequentially when invoked. This feature enables event handling scenarios where multiple event handlers can be attached to an event.