- Interview Preparation
- C# Interview Questions
- MVC Interview Questions
- Learning
- Learn C#
- Tools
- JSON Formatter & Minifier
- XML Formatter & Minifier
- CSS Formatter & Minifier
- SQL Formatter & Minifier
- Online Editors
- C# Editor
- HTML Editor
Top MVC Interview Questions and Answers for 2025
Introduction
Are you planning to prepare for MVC technical interview? In this article, we will discuss top MVC Interview Questions and Answers which are suitable and relevant for freshers, intermediate and experienced professionals. You can prepare all latest MVC interview questions for 2025. These MVC interview questions are written under the guidance of the masters of MVC and by getting ideas through the experience of employees recent MVC coding interviews.
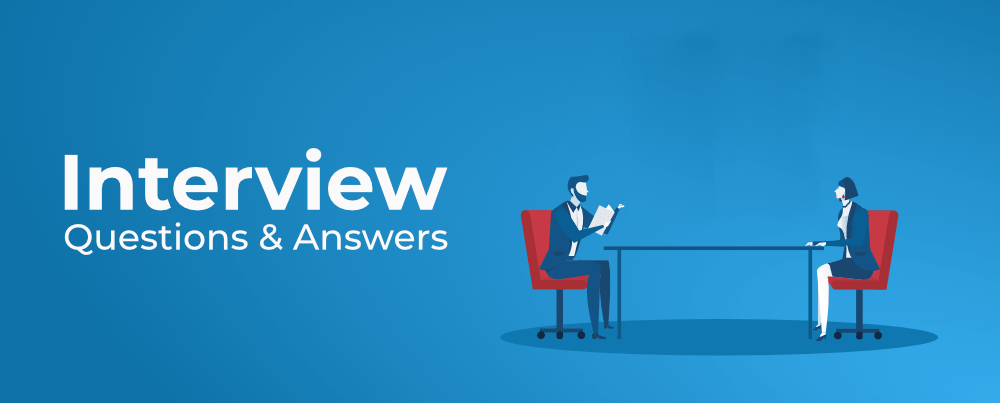
MVC Interview Questions & Answers
1. What is ASP.NET MVC?
ASP.NET MVC is a web application framework developed by Microsoft based on the MVC architectural pattern. It provides a structured approach for building dynamic, data-driven web applications using the Model-View-Controller pattern.
ASP.NET MVC provides a structured and flexible approach to building web applications, allowing developers to create clean, maintainable code that is easily testable. It promotes separation of concerns, making it easier to manage different aspects of an application independently. Additionally, ASP.NET MVC offers features such as routing, which enables clean and customizable URLs, and built-in support for dependency injection, facilitating better code organization and maintainability.
Overall, ASP.NET MVC is a powerful framework for developing modern, scalable web applications, offering developers the tools they need to build robust and efficient solutions.
2. Explain the role of each component in MVC: Model, View, and Controller
- Model: The Model represents the application's data and business logic. It encapsulates the application's state and behavior, interacting with the database or other data sources.
- View: The View is responsible for presenting the application's user interface. It displays data from the Model to the user and handles user interactions, such as submitting forms or clicking buttons.
- Controller: The Controller acts as an intermediary between the Model and View. It receives user input, processes it, interacts with the Model to perform any necessary operations (such as retrieving or updating data), and then selects the appropriate View to display the results to the user.
3. What are the advantages of using MVC?
Using the MVC (Model-View-Controller) architectural pattern offers several advantages in software development.
- Separation of Concerns: MVC separates different aspects of an application, such as data management, user interface, and business logic, into distinct components (Model, View, and Controller). This separation allows for cleaner and more organized code, making it easier to maintain and update the application over time.
- Modularity: The modular structure of MVC enables developers to work on different parts of the application independently. This promotes code reusability, as well as easier collaboration among team members working on different aspects of the project.
- Improved Testability: With MVC, each component can be unit tested in isolation, making it easier to identify and fix bugs. By separating concerns, developers can write more focused and comprehensive tests for each part of the application, leading to higher code quality and reliability.
- Flexibility: MVC provides flexibility in terms of technology choices and development approaches. Developers can choose the most suitable technologies and frameworks for each component, allowing for greater customization and adaptability to project requirements.
- Enhanced Maintainability: Due to its modular and organized structure, applications built using MVC are typically easier to maintain and extend. Changes to one component, such as updating the user interface or modifying business logic, can be made without affecting other parts of the application, reducing the risk of unintended side effects.
- Scalability: MVC architecture scales well with the growth of an application. As the application becomes more complex or requires additional features, developers can easily add or modify components without restructuring the entire application, thus ensuring scalability and future-proofing.
4. Explain the MVC request processing lifecycle in ASP.NET MVC
MVC lifecylce contains 4 stages to process a request.
- Routing: Incoming requests are mapped to controller actions based on routing configuration. Routing gives you a clean url which is easy to read.
- Controller Execution: Based on routing configuration, the appropriate controller is selected, and the corresponding action method is executed.
- Action Execution: The action method performs the required processing, typically involving interactions with the model layer.
- View Rendering: The controller passes data to the view, and the view is rendered to generate the HTML response.
5. Explain the concept of routing in ASP.NET MVC and how it works
Routing in ASP.NET MVC is the process of matching incoming URL requests to controller action methods. It determines how incoming requests are handled and which controller and action method should be invoked to generate a response. The routing engine then parses incoming URLs, identifies the matching route, and invokes the corresponding controller action.
In simpler terms, routing acts as a traffic director for web requests in an MVC application. When a user navigates to a specific URL, the routing mechanism determines which controller should handle the request and which action method within that controller should be executed.
For example, consider a URL like "example.com/home/index". The routing system would interpret this URL to mean that the "index" action method within the "home" controller should be invoked to generate the corresponding webpage.
6. What is attribute-based routing in ASP.NET MVC?
Attribute-based routing is a feature introduced in ASP.NET MVC 5 that allows developers to define routes using attributes applied directly to controller action methods. It provides a more declarative and intuitive way to specify routing rules, improving code readability and maintainability.
7. Explain the concept of routing constraints in ASP.NET MVC
Routing constraints in ASP.NET MVC are used to restrict the values that can be matched by route parameters in route definitions. Constraints are defined using regular expressions or custom constraint classes and are applied to route parameters to ensure that only values that satisfy the specified criteria are accepted. Constraints help to create more specific and predictable route configurations.
In simple terms, routing constraints act as filters for incoming requests, allowing only those that meet certain conditions to be handled by the corresponding controller actions. This helps to improve the accuracy and efficiency of routing in ASP.NET MVC applications by ensuring that only valid URLs are processed.
For example, if you have a route parameter that should only match numeric values, you can apply a constraint to ensure that only numeric inputs are accepted. Similarly, you can define constraints for other types of inputs such as strings, dates, or even custom patterns.
8. Explain the role of the RouteConfig class in ASP.NET MVC
The RouteConfig class is responsible for defining and configuring routes in an ASP.NET MVC application. It typically resides in the App_Start folder and is invoked during application startup to register route mappings using the RouteTable.Routes collection.
For example
using System.Web.Mvc;
using System.Web.Routing;
namespace ProjectName
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
// Default route
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}
}
}
In this example:
The RegisterRoutes method is responsible for defining the routes.
The IgnoreRoute method is used to exclude certain URLs from being processed by MVC. In this case, requests for .axd resources are ignored.
The MapRoute method defines a default route for the application. It specifies the route pattern {controller}/{action}/{id}, where controller and action represent the controller and action method names, respectively. The id parameter is optional and can be omitted from the URL. If no controller or action is specified in the URL, the default values "Home" and "Index" are used.
9. Explain the role of the Web.config file in ASP.NET MVC
The Web.config file is a configuration file in ASP.NET MVC used to configure various settings and parameters for the application, including connection strings, application settings, custom error handling, session state management, authentication, authorization, and other runtime behaviors.
10. What are the different types of ActionResult return types in ASP.NET MVC?
There are different types of action results in ASP.NET MVC. Each result has a different type of result format to view page. Here's a breakdown of the most common ones:
- ViewResult: ViewResult represents a result that renders a view to the response. It will return an HTML so we can display in view. View result can return data to view page through which class is defined in the model. view page has “.cshtm” extension.
- PartialViewResult: Similiar to ViewResult, but specifically used to return partial view. PartialView typically used to render a view inside a normal view. We should create a Partial view inside shared folder, otherwise we cannot access the Partial view.
- RedirectResult: Represents a result that performs an HTTP redirect to a specified URL. Used to redirect the user to a different action or external URL. If it gives wrong URL, it will show 404 page errors.
- RedirectToAction: RedirectToActionResult is returning the result to a specified controller and action method.
- JsonResult: Return a result that serializes data into JSON format and returns it to the client. Used for AJAX requests or returning structured data to JavaScript clients.
- FileResult: Return a result that returns a file to the client. Can be used to return various file types such as images, PDFs, or Excel spreadsheets.
- ContentResult: Content result returns different content's format to view. MVC returns different format using content return like HTML format, Java Script format and any other format.
11. What is the purpose of the JsonResult return type in ASP.NET MVC, and how is it used?
The JsonResult return type in ASP.NET MVC is used to return JSON-formatted data from controller actions. It serializes the specified data object to JSON format and sends it as the response to the client. JsonResult is commonly used in AJAX-based scenarios where data needs to be exchanged between the client and server in JSON format.
12. Explain the concept of partial views in ASP.NET MVC, and how are they used?
Partial views in ASP.NET MVC are reusable view components that can be rendered within other views or layout views. They are similar to user controls in Web Forms and allow for the encapsulation of UI components into separate files. Partial views are commonly used to render reusable UI elements such as navigation menus, sidebars, or widgets, and promote code reusability and modularity. We should create a Partial view inside shared folder, otherwise we cannot access the Partial view.
13. What is ViewBag, ViewData, and TempData in ASP.NET MVC?
ViewBag, ViewData, and TempData are mechanisms for passing data from controllers to views.
- ViewBag: Dynamic property bag used to pass data from the controller to the view.
- ViewData: Dictionary-like object used to pass data from the controller to the view.
- TempData: Dictionary-like object used to pass data between controller actions during the same request or across redirects.
14. Explain the difference between TempData and Session in ASP.NET MVC
TempData is used to pass data between controller actions during the same request or across redirects and is available only for the duration of the current and subsequent requests. Session, on the other hand, is a server-side storage mechanism that persists data across multiple requests and user sessions.
15. Explain the role of a layout view in ASP.NET MVC
A layout view in ASP.NET MVC provides a consistent layout structure across multiple views by defining common UI elements such as headers, footers, and navigation menus. It reduces redundancy in view code and promotes code reusability.
16. What is the purpose of HTML Helpers in ASP.NET MVC?
HTML Helpers are methods that generate HTML markup programmatically in ASP.NET MVC views. They simplify the process of generating HTML elements and help maintain clean and concise view code.
17. What is model binding in ASP.NET MVC?
Model binding is the process of mapping HTTP request data to action method parameters or model properties in ASP.NET MVC. It automatically populates model properties based on form data, query string values, or route data.
18. What is a ViewModel in ASP.NET MVC?
A ViewModel is a class used to represent the data and behavior required by a view in ASP.NET MVC. It encapsulates the data necessary for rendering a view and may include additional properties or methods tailored to the specific view's requirements.
19. Explain the role of filters in ASP.NET MVC
Filters are used to perform the custom logic before or after executing the action method. Filters are attributes applied to controllers or action methods in ASP.NET MVC to modify the behavior of action execution, such as authentication, authorization, logging, caching, and exception handling. They provide a way to encapsulate cross-cutting concerns and promote code reuse.
20. What are the different types of action filters in ASP.NET MVC?
Common types of action filters include:
- Action Filter: Action filters are executed before and after an action method is executed. They allow you to perform additional processing such as logging, validation, or modifying the result returned by the action method. Examples include ActionFilterAttribute, OutputCacheAttribute, and ValidateAntiForgeryTokenAttribute.
- Authentication Filter: Authentication filter is introduced with ASP.NET MVC5. It authenticates the credentials of a user like a username and password. IAuthenticationFilter interface is used to create CustomAuthentication filter.
- Authorization Filter: These filters determine whether a user is authorized to access a particular action method or controller. Examples include AuthorizeAttribute and AllowAnonymousAttribute.
- Result Filter: Result filters are executed before and after the result of an action method is executed. They allow you to modify the result returned by the action method or perform additional processing such as logging or caching. Examples include ResultFilterAttribute.
- Exception Filter: Exception filters handle exceptions that occur during the execution of an action method. They allow you to perform custom exception handling logic such as logging errors or displaying error messages to users.
21. What is the function of beforeRender() in the controller?
This function is required when we call render() manually before the end of a given action. This function is called before the view is rendered and after controller action logic. It is not used often.
22. What do you know about beforeFilter() and afterFilter() functions in controllers?
beforeFilter() is run every action in the controller. It is the right situation to inspect permissions or check for an active session. At the same time, afterFilter() is called after every rendering is done and after every controller action. This is the last controller method to be run.
23. What is the difference between ‘ViewResult’ and ‘ActionResult’?
ViewResult is derived from the 'AbstractResult' class, and 'ActionResult’ is an abstract class. ActionResult is good when you are dynamically deriving different types of views. The derived classes of ActionResult are FileStreamResult, ViewResult, and JsonResult.
24. Define POST and GET action types
POST action type submits data to be processed to a specified resource. We pass the essential URL and data with all the POST requests. It can take up overloads. POST action used when you have large and sensitive data to send .
GET action type requests data from a specified resource. We pass the compulsory URLs with all the GET requests. It can take up overloads. GET action type carry all data in query string.
25. Explain the concept of Areas in ASP.NET MVC
Areas in ASP.NET MVC allow developers to partition large web applications into smaller, self-contained modules based on functional areas or feature sets. Each area can have its own controllers, views, models, and other resources, providing a structured way to organize and manage complex applications.
For example, if you want to seperate user portal and admin portal then we can create two areas and both areas will have their own controllers, views, models and other resources.
26. Explain the role of the @Html.Partial and @Html.RenderPartial methods in ASP.NET MVC views
@Html.Partial and @Html.RenderPartial are HTML helper methods used to render a partial view within another view in ASP.NET MVC.
@Html.Partial: Renders the partial view synchronously and returns the HTML markup as a string.
@Html.RenderPartial: Renders the partial view directly to the response stream and does not return any HTML markup. It is more efficient for rendering large partial views.
27. What is Dependency Injection (DI) in ASP.NET MVC, and why is it used?
Dependency Injection (DI) is a design pattern used to manage object dependencies and promote loose coupling between components in ASP.NET MVC applications. It allows objects to receive their dependencies from an external source rather than creating them internally, making the code more modular, testable, and maintainable.
28. Explain the role of the Global.asax file in ASP.NET MVC
The Global.asax file serves as the application-level configuration file in ASP.NET MVC. It contains application-level events and handlers, such as Application_Start and Application_Error, which are executed during application startup, shutdown, and error handling. It also provides a central location for configuring global application settings and registering application-level components.
29. What is the purpose of the @Html.Action and @Html.RenderAction methods in ASP.NET MVC views?
@Html.Action and @Html.RenderAction are HTML helper methods used to invoke child actions within a view in ASP.NET MVC.
@Html.Action: Invokes a child action synchronously and includes the resulting HTML markup in the parent view.
@Html.RenderAction: Invokes a child action directly to the response stream and does not return any HTML markup. It is more efficient for rendering child actions.
30. Explain the concept of bundling and minification in ASP.NET MVC
Bundling and minification are techniques used to improve the performance of web applications by reducing the number of HTTP requests and minimizing the size of CSS and JavaScript files.
Bundling: Groups multiple CSS or JavaScript files into a single bundle, reducing the number of requests required to load resources.
Minification: Minifies CSS and JavaScript files by removing unnecessary whitespace, comments, and renaming variables to shorten code size, resulting in faster downloads and improved page load times.
31. How will you implement validation in MVC?
We can implement validation in the MVC application with the help of validators defined in the System.ComponentModel.DataAnnotations namespace. The different validators are DataType, Required, Range, and StringLength.
32. What is the purpose of the ModelState.IsValid property in ASP.NET MVC?
The ModelState.IsValid property is used to check whether the model state is valid after model binding in ASP.NET MVC. It returns true if no validation errors are found in the model state, indicating that the input provided by the user is valid and can be processed further.
33. Explain the difference between TempData.Peek and TempData["key"] in ASP.NET MVC
TempData.Peek and TempData["key"] are both used to access data stored in TempData in ASP.NET MVC. TempData.Peek: Retrieves the value associated with the specified key from TempData without removing it from the collection. If the key does not exist, it returns null. TempData["key"]: Retrieves the value associated with the specified key from TempData and removes it from the collection. If the key does not exist, it returns null.
34. What is the purpose of the Authorize attribute in ASP.NET MVC?
The Authorize attribute is used to restrict access to controller actions or entire controllers in ASP.NET MVC based on user authentication and authorization. It ensures that only authenticated users with specific roles or permissions can access protected resources, redirecting unauthenticated users to the login page by default.
35. What is the role of the AntiForgeryToken in ASP.NET MVC, and why is it used?
The AntiForgeryToken is a security feature in ASP.NET MVC used to prevent Cross-Site Request Forgery (CSRF) attacks. It generates a unique token for each form submission, which is validated on the server to ensure that the request originates from the genuine application view and not from a malicious source.
36. Explain the concept of scaffolding in ASP.NET MVC
Scaffolding is a code generation technique in ASP.NET MVC used to generate boilerplate code for common CRUD (Create, Read, Update, Delete) operations based on a data model. It automatically generates controller actions, views, and data access code to interact with the underlying data source, speeding up development and reducing manual coding effort.
37. Explain the concept of model binding in ASP.NET MVC
Model binding is the process of mapping HTTP request data to action method parameters or model properties in ASP.NET MVC. It automatically populates model properties based on form data, query string values, or route data, simplifying the process of extracting data from incoming requests.
38. Explain the concept of HTML Helpers in ASP.NET MVC, and provide examples of different types of HTML Helpers
HTML Helpers are methods in ASP.NET MVC used to generate HTML markup programmatically in views. They simplify the process of creating HTML elements and provide strongly-typed alternatives to traditional HTML markup. Examples of HTML Helpers include TextBoxFor, DropDownListFor, CheckBoxFor, LabelFor, ActionLink, and Partial.
39. What is the purpose of the @section directive in Razor views in ASP.NET MVC?
The @section directive in Razor views is used to define named sections of content that can be overridden or replaced by child views. It allows for modularization of view content and facilitates the creation of reusable layouts and templates. @section directives are commonly used in conjunction with layout views to define content areas such as scripts, stylesheets, or page titles.
40. What is the purpose of the @helper directive in Razor views in ASP.NET MVC?
The @helper directive in Razor views is used to define reusable code snippets or helper methods that can be called from within the view. It allows for the encapsulation of common rendering logic into modular components, improving code reuse and maintainability. @helper methods are commonly used for generating repetitive HTML markup or performing data formatting tasks within views.
41. What do you understand by ViewState in MVC?
One of the most common asp net interview questions is based on ViewState. Unlike WebForms, MVC does not have ViewState. This is because ViewState is stored in a hidden field on the page, which increases its size significantly and impacts the page loading time.